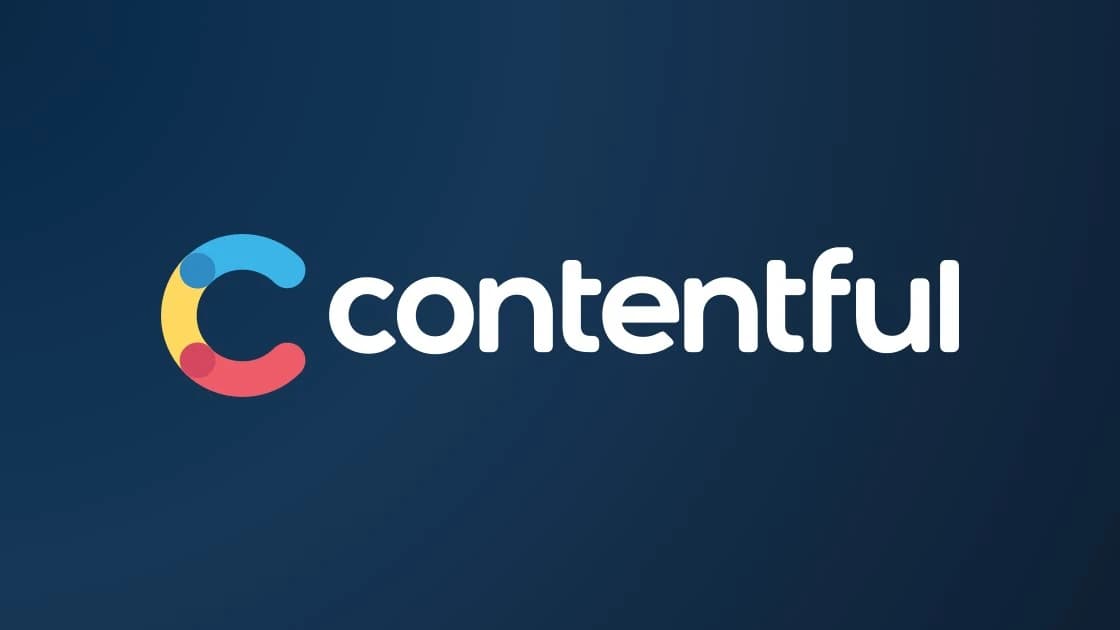
Building Scalable Apps with Contentful CMS
Phyo Zin Wai / March 21, 2025
Why Contentful CMS?
As a developer, choosing the right CMS can make or break a project. Contentful is a headless CMS that offers flexibility, scalability, and API-driven content management, making it ideal for both websites and mobile apps.
Unlike traditional CMS platforms that tightly couple content and presentation, Contentful decouples these aspects, allowing developers to build frontend applications in any framework while using Contentful purely for content storage and management.
Key Features
1. API-First Approach
Contentful provides powerful REST and GraphQL APIs, allowing developers to fetch and manage content seamlessly across platforms. The GraphQL API is particularly useful for reducing over-fetching, as it lets you request only the fields you need.
Common Problem: Over-Fetching Data
With REST APIs, you often receive more data than needed, slowing down the application. Contentful's GraphQL API solves this by allowing you to request only the required fields.
query {
blogPostCollection {
items {
title
description
author {
name
}
}
}
}
2. Content Modeling and Structuring
With Contentful’s UI, you can define custom content types, set relationships between entries, and manage assets like images and videos with ease. However, one common challenge is designing an efficient content model that balances flexibility with structure.
Common Problem: Poor Content Modeling
If you don't plan your content model properly, you may face issues with scalability. For example, storing all blog post data in a single "rich text" field may work for small projects, but for larger applications, breaking it into separate fields (title, summary, body, metadata) improves performance and content reusability.
3. Multi-Platform Support
Since Contentful is a headless CMS, the same content can be delivered to websites, mobile apps (iOS/Android), and even IoT devices. This means you can maintain a single source of truth for your content, reducing duplication and inconsistencies.
Common Problem: Handling Localization
If you need to serve content in multiple languages, Contentful provides built-in localization support. However, managing translations manually can be tedious. A better approach is to use Contentful’s localization API to programmatically update translations.
const entry = await client.getEntry('your_entry_id');
console.log(entry.fields.title['en-US']); // Fetch English version
console.log(entry.fields.title['fr-FR']); // Fetch French version
Integrating Contentful with Next.js
For a seamless experience, integrating Contentful with Next.js ensures efficient data fetching and static site generation (SSG). This approach significantly improves page load speeds and SEO.
Installation
npm install contentful
Fetching Data in Next.js
The most efficient way to fetch data is using getStaticProps
to generate static pages at build time, reducing API calls on runtime.
import { createClient } from 'contentful';
const client = createClient({
space: process.env.CONTENTFUL_SPACE_ID,
accessToken: process.env.CONTENTFUL_ACCESS_TOKEN,
});
export async function getStaticProps() {
const res = await client.getEntries({ content_type: 'blogPost' });
return { props: { posts: res.items }, revalidate: 60 };
}
Common Problem: Handling Dynamic Content Updates
Since static generation means data is fetched at build time, updates in Contentful won’t immediately reflect on the site. To fix this, use Incremental Static Regeneration (ISR) by adding revalidate
, so the page regenerates at regular intervals.
Deploying Contentful-powered Apps
Once your app is ready, you can deploy it to platforms like Vercel, Netlify, or AWS. Contentful ensures content is always up-to-date without requiring code changes.
Common Pitfall: Managing Content in Staging vs Production
A common mistake is modifying live content during testing. Contentful provides "Environments" to create staging versions of your content. You can switch environments in your API calls to ensure proper testing before pushing updates live.
const client = createClient({
space: process.env.CONTENTFUL_SPACE_ID,
accessToken: process.env.CONTENTFUL_ACCESS_TOKEN,
environment: 'staging',
});
Conclusion
Contentful CMS is a game-changer for developers looking to build modern, scalable, and API-driven websites and mobile applications. Whether you’re working on a startup or an enterprise-grade application, Contentful’s flexibility makes it an excellent choice.
However, proper planning is crucial to avoid common pitfalls such as poor content modeling, over-fetching data, and content version management. By leveraging Contentful’s APIs, content structuring best practices, and Next.js integration, you can build a robust and efficient content-driven application.